Project Overview:
The Personal Expense Tracker is a Python-based application that allows users to input their daily expenses, categorize them (e.g., food, transport, entertainment), and generate a summary report of their spending over time. The application also includes a simple user interface using tkinter
and stores data in a SQLite database.
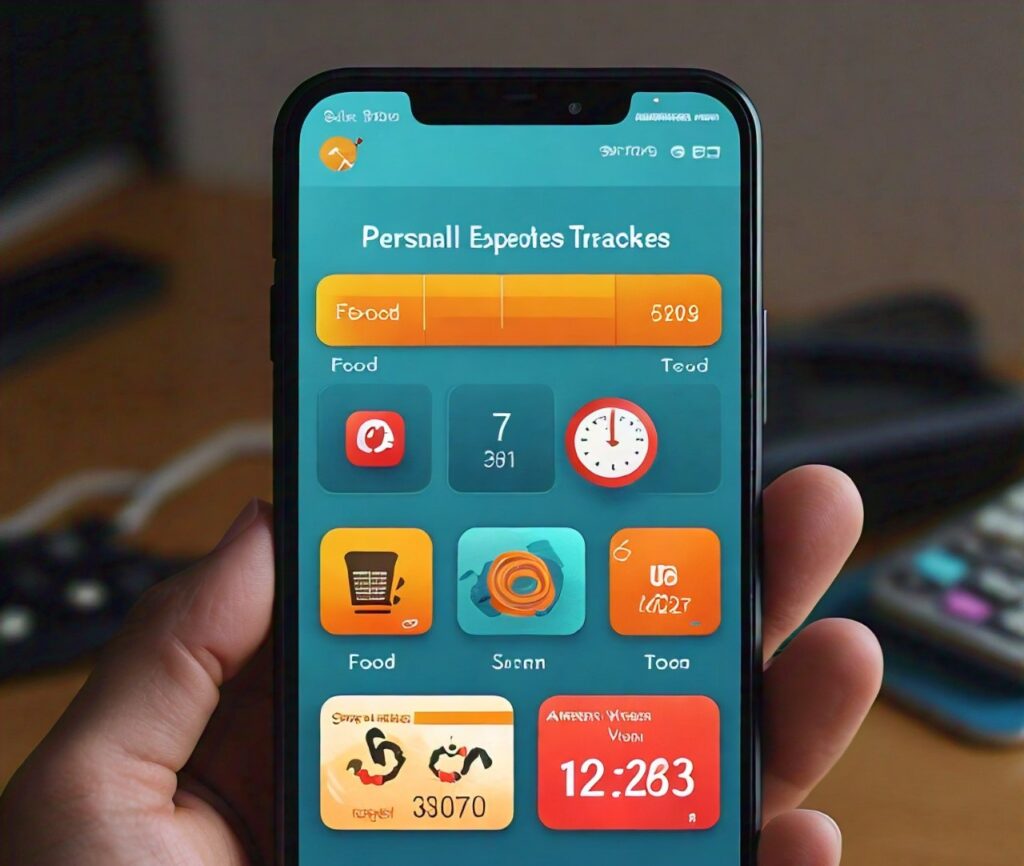
Features:
- Add Expense: Users can add expenses with a description, category, amount, and date.
- View Expenses: Display a list of all expenses with filtering options by date and category.
- Generate Report: Provide a summary of expenses over a selected period, showing total spent per category.
- Data Storage: Use SQLite to store expenses persistently.
Code Implementation:
Step 1: Set Up the SQLite Database
import sqlite3
def create_database():
conn = sqlite3.connect('expenses.db')
c = conn.cursor()
c.execute('''
CREATE TABLE IF NOT EXISTS expenses (
id INTEGER PRIMARY KEY,
description TEXT,
category TEXT,
amount REAL,
date TEXT
)
''')
conn.commit()
conn.close()
create_database()
Step 2: Add Expense Functionality
def add_expense(description, category, amount, date):
conn = sqlite3.connect('expenses.db')
c = conn.cursor()
c.execute('''
INSERT INTO expenses (description, category, amount, date)
VALUES (?, ?, ?, ?)
''', (description, category, amount, date))
conn.commit()
conn.close()
Step 3: View Expenses Functionality
def view_expenses(filter_category=None, filter_date=None):
conn = sqlite3.connect('expenses.db')
c = conn.cursor()
query = "SELECT * FROM expenses WHERE 1=1"
params = []
if filter_category:
query += " AND category = ?"
params.append(filter_category)
if filter_date:
query += " AND date = ?"
params.append(filter_date)
c.execute(query, params)
expenses = c.fetchall()
conn.close()
for expense in expenses:
print(expense)
Step 4: Generate Expense Report
def generate_report():
conn = sqlite3.connect('expenses.db')
c = conn.cursor()
c.execute('''
SELECT category, SUM(amount) FROM expenses
GROUP BY category
''')
report = c.fetchall()
conn.close()
print("Expense Report:")
for category, total in report:
print(f"{category}: ${total:.2f}")
Step 5: User Interface with tkinter
import tkinter as tk
from tkinter import messagebox
def add_expense_ui():
def submit():
description = desc_entry.get()
category = category_entry.get()
amount = float(amount_entry.get())
date = date_entry.get()
add_expense(description, category, amount, date)
messagebox.showinfo("Success", "Expense added successfully!")
window.destroy()
window = tk.Tk()
window.title("Add Expense")
tk.Label(window, text="Description").grid(row=0)
tk.Label(window, text="Category").grid(row=1)
tk.Label(window, text="Amount").grid(row=2)
tk.Label(window, text="Date (YYYY-MM-DD)").grid(row=3)
desc_entry = tk.Entry(window)
category_entry = tk.Entry(window)
amount_entry = tk.Entry(window)
date_entry = tk.Entry(window)
desc_entry.grid(row=0, column=1)
category_entry.grid(row=1, column=1)
amount_entry.grid(row=2, column=1)
date_entry.grid(row=3, column=1)
tk.Button(window, text="Submit", command=submit).grid(row=4, column=1)
window.mainloop()
def main_ui():
window = tk.Tk()
window.title("Expense Tracker")
tk.Button(window, text="Add Expense", command=add_expense_ui).pack()
tk.Button(window, text="View Expenses", command=view_expenses).pack()
tk.Button(window, text="Generate Report", command=generate_report).pack()
window.mainloop()
main_ui()
How to Run the Project:
- Save the code in a Python file (e.g.,
expense_tracker.py
). - Install required libraries if not already installed:
pip install tkinter
- Run the Python file:
python expense_tracker.py
Project Presentation:
- Introduction: Explain the problem statement and how your project solves it.
- Demonstration: Walk through the code and show how to add expenses, view them, and generate reports.
- Conclusion: Summarize the key features and future improvements you could make (e.g., adding charts, exporting reports as CSV).
This project not only showcases your Python skills but also demonstrates practical application development.
Leave a Reply